This class implements a rigid-tendon muscle-torque-generator (MTG) for a growing list of joints and torque-directions. For a detailed description of the MTGs available and the automatic fitting routine (implemented in TorqueMuscleFittingToolkit) please see the publication: M.Millard, A.L.Kleesattel, M.Harant, & K.Mombaur. A reduced muscle model and planar musculoskeletal model fit for the synthesis of whole body movements. Journal of Biomechanics. (under review as of August 2018)
More...
|
void | updateTorqueMuscleCurves () |
|
double | calcJointAngle (double fiberAngle) const |
|
double | calcFiberAngle (double jointAngle) const |
|
double | calcFiberAngularVelocity (double jointAngularVelocity) const |
|
double | calcJointAngularVelocity (double fiberAngularVelocity) const |
|
void | calcTorqueMuscleDataFeatures (RigidBodyDynamics::Math::VectorNd const &jointTorque, RigidBodyDynamics::Math::VectorNd const &jointAngle, RigidBodyDynamics::Math::VectorNd const &jointAangularVelocity, double activeTorqueAngleBlendingVariable, double passiveTorqueAngleBlendingVariable, double torqueVelocityBlendingVariable, double activeTorqueAngleAngleScaling, double activeTorqueAngleAtOneNormTorque, double passiveTorqueAngleCurveOffset, double maxAngularVelocity, double maxActiveIsometricTorque, TorqueMuscleDataFeatures &tmf) const |
|
double | calcFiberAngleGivenNormalizedPassiveTorque (double normPassiveFiberTorque, double blendingVariable, double passiveTorqueAngleCurveOffset) const |
|
double | calcBlendedCurveDerivative (double curveArgument, double blendingVariable, double maximumBlendingValue, unsigned int derivativeOrderArgument, unsigned int derivativeOrderBlendingVariable, RigidBodyDynamics::Addons::Geometry::SmoothSegmentedFunction const &curve) const |
|
double | calcInverseBlendedCurveValue (double blendedCurveValue, double argGuess, double blendingVariable, double maximumBlendingValue, RigidBodyDynamics::Addons::Geometry::SmoothSegmentedFunction const &curve) const |
|
void | updTorqueMuscleSummary (double activation, double jointAngle, double jointAngularVelocity, double activeTorqueAngleBlendingVariable, double passiveTorqueAngleBlendingVariable, double activeTorqueAngularVelocityBlendingVariable, double activeTorqueAngleAngleScaling, double activeTorqueAngleAtOneNormTorque, double passiveTorqueAngleCurveOffset, double maxAngularVelocity, double maxActiveIsometricTorque, TorqueMuscleSummary &updTms) const |
|
void | updTorqueMuscleSummaryCurveValues (double fiberAngle, double normFiberAngularVelocity, double activeTorqueAngleBlendingVariable, double passiveTorqueAngleBlendingVariable, double activeTorqueAngularVelocityBlendingVariable, double activeTorqueAngleAngleScaling, double activeTorqueAngleAtOneNormTorque, double passiveTorqueAngleCurveOffset, TorqueMuscleSummary &updTms) const |
|
void | updTorqueMuscleInfo (double activation, double jointAngle, double jointAngularVelocity, double activeTorqueAngleBlendingVariable, double passiveTorqueAngleBlendingVariable, double activeTorqueAngularVelocityBlendingVariable, double activeTorqueAngleAngleScaling, double activeTorqueAngleAtOneNormTorque, double passiveTorqueAngleCurveOffset, double maxAngularVelocity, double maxActiveIsometricTorque, TorqueMuscleInfo &updTmi) const |
|
void | updInvertTorqueMuscleSummary (double jointTorque, double jointAngle, double jointAngularVelocity, double activeTorqueAngleBlendingVariable, double passiveTorqueAngleBlendingVariable, double activeTorqueAngularVelocityBlendingVariable, double activeTorqueAngleAngleScaling, double activeTorqueAngleAtOneNormTorque, double passiveTorqueAngleCurveOffset, double maxAngularVelocity, double maxActiveIsometricTorque, TorqueMuscleSummary &updTms) const |
|
This class implements a rigid-tendon muscle-torque-generator (MTG) for a growing list of joints and torque-directions. For a detailed description of the MTGs available and the automatic fitting routine (implemented in TorqueMuscleFittingToolkit) please see the publication: M.Millard, A.L.Kleesattel, M.Harant, & K.Mombaur. A reduced muscle model and planar musculoskeletal model fit for the synthesis of whole body movements. Journal of Biomechanics. (under review as of August 2018)
This rigid-tendon torque muscle model provides modeling support for 3 phenomena
- torque-angle curve (
): the variation of active isometric torque in one direction as a function of joint angle
- torque-velocity curve (
): the variation of torque as a function of angular velocity
- passive-torque-angle curve (
): the variation of passive torque as a function of joint angle. Here
and
are user-defined scaling and shift parameters.
each of which are represented as smooth normalized curves that vary between 0 and 1. A series of scaling (
), shifting (
), and blending (
) variables have been introduced to these curves to make it possible to easily fit these curves to a specific subject. These fitting variables can be set/fitted by making use of the functions in this class. Alternatively these fitting variables can be automatically adjusted (using IPOPT) by making use of the TorqueMuscleFittingToolkit.
Examples of the adjustable characteristic curves
These three curves are used to compute the torque developed
given the angle of the joint
, the angular-velocity of the joint
, and the activation of the muscle
(a 0-1 quantity that defines how much the muscle is turned-on, or activated), and the maximum-isometric torque
The damping term
is necessary to supress vibration that will occur as the passive element
is streched, its stiffness increases, and the natural frequency of the overall system rises. By default
is set to 0.1 which has proven effective for supressing vibration in the trunk segments during a stoop lift in which the stiffness of the lumbar back muscles grows appreciably. This model does not yet provide support for the following phenomena but will in the future.
- activation dynamics: currently is left to the user
- tendon-elasticity
- muscle short-range-stiffness
All of these characteristic curves are represented using
continuous
order Bezier curves that have been fitted to the data from data in the literature. In many cases these curves have been carefully edited so that they fit the curves of the original papers, but have more desireable numerical properties for optimal control work. The characterisic curves provided by this class have been fitted to a growing list of data sets: -Anderson Data Set: from Anderson et al. 2007 -Whole-body Gymnast Data Set: from Jackson, Kentel et al., Anderson et al., Dolan et al. and Raschke et al.
Data Set: Anderson2007
This data set uses the mean value of the coefficients published in Anderson et al. The standard deviation table has also been entered. However, since it is unclear how to use the standard deviation in a consistent way across all joints/parameters this table is not yet accessible through the constructor. This data set includes coefficients for the following
- Number of subjects: 34
- Gender: male and female
- Age: young (18-25, 14 subjects), middle-aged (55-65, 14 subjects), senior (> 65, 6 subjects)
- Joint: hip/knee/ankle
- Direction: extension/flexion
Notes
- Angles are plotted using units of degrees for readability. The actual curves are described in units of radians
- See Anderson et al. for further details.
Characteristic from Anderson et al. 2007 [1]
Data Set: Gymnast
This data set is an attempt at making enough torque muscles for a whole body. Since no single source in the literature comes close to measuring the characteristics of all of the joints, data from Jackson et al., Kentel et al., Anderson et al., Dolan et al, and Raschke et al. have been combined. Since the subjects used in these various studies are wildly different (Jackson et al. measured an elite male gymnast; Kentel et al. measured an elite tennis player; Anderson et al. measured, in the category of young male, a selection of active undergraduate students, Dolan et al from 126 women and 23 men, and Raschke from 5 male subjects) scaling has been used to make the strength of the subject consistent. Scaling coefficients for the lower body, shoulders and elbow, and forearm/wrist have been calculated using measurements that overlapped between datasets. Presently this data set includes curves for 22 torque muscles.
- Number of subjects: 1 elite gymnast (69.6 kg, 1.732 m)
- Gender: male
- Age: 21 years old
- Joint and Directions available
- Ankle: flexion/extension (scaled from Anderson)
- Knee: flexion/extension (from Jackson)
- Hip: flexion/extension (from Jackson)
- Lumbar: active extension curves (
and
) from Raschke et al. passive extension from Dolan et al. The torque velocity curve has since been updated using an estimate from the archiecture of the lumbar extensors
- Lumbar: active flexion
from Beimborn et al.
- Shoulder: flexion/extension (from Jackson)
- Shoulder: horizontal adduction/abduction (from Kentel, scaled to Jackson's subject)
- Shoulder: internal rotation/external rotation (from Kentel, scaled to Jackson's subject)
- Elbow: flexion/extension (from Kentel, scaled to Jackson's subject)
- Wrist: pronation/supination (from Kentel, scaled to Jackson's subject)
- Wrist: extension/flextion (from Jackson)
- Wrist: ulnar/radial deviation (from Kentel, scaled to Jackson's subject)
- Missing Joint and directions
- Ankle inversion/eversion
- Hip adduction/abduction
- Hip internal rotation/external rotation
- Lumbar extension/flexion
- Lumbar bending
- Lumbar twisting
- Shoulder Adduction
- Shoulder Abduction
- Scapular elevation/depression
- Scapular adduction/abduction
- Scapular upward/downward rotation
In all cases the curves have been fitted to Bezier curves that are constructed using functions in TorqueMuscleFunctionFactory.
Notes
- Angles are plotted using units of degrees for readability. The actual curves are described in units of radians
- Hip and Knee characteristics taken from Jackson. Ankle extension is from Anderson et al., scaled using Jackson-to-Anderson hip/knee strength ratios from Jackson ratios
- Shoulder horizontal adduction/abduction and internal/external rotation is a scaled version of the Kentel. Strength was scaled using the Jackson-to-Kentel shoulder flex/ext ratios.
- Elbow extension/flexion and forearm pronation/supination. Elbow strength scaled from Kentel using the ratio of maximum isometric shoulder ext/flextion between Kentel and Jackson. Forearm pronation/supination scaled using the maximum torque strength ratio of wrist extension/flextion between Kentel and Jackson
- Wrist ext/flexion directly from Jackson, while the curves for ulnar and radial deviation have been scaled (using the maximum isometric torque ratios of wrist extension and flexion from both models) from Kentel et al.
- Lumbar-extension active-torque-angle-curve in extension comes from Raschke et al.
- Lumbar-extension passive-torque-angle-curve comes from Dolan et al.
- Lumbar-flexion
comes from Beimborn et al.'s observation that the strength ratio of back extensors to flextors at a flextion angle of zero is most often repored as 1.3:1.
- Lumbar-extension and flexion torque-velocity-curves have been estumated using the architecture of these two muscles. This update has been made because the force-velocity curve proposed by Raschke and Chaffin in Fig. 4 has such a low maximum angular velocity (60 dec/sec) that none of our optimal control simulations predicted lumbar flexion during movement. Using muscle archectural information for the erector spinae (ES) and rectus abdominus (RA), assuming both are made of slow twitch fibers the maximum angular velocites are 433 deg/sec and 1102 deg/sec about the constant assumed moment arms for the lumbar extensors and flexors respectively. The following architectural information was used:
- 7.1 cm :ES moment arm from Németh & Ohlsén
- 8.08 cm :ES optimal fiber length taken from a weighted PSCA average of the ES muscles from Christophy et al. Table 1
- 7.02
:ES maximum angular velocity for slow twitch muscle from Ranatunga
- 0.151 :ES tv at half max. angular velocity
- 10.9 cm :RA moment arm from Németh & Ohlsén
- 29.9 cm :RA optimal fiber length from Christophy et al. Table 1
- 7.02
:RA maximum angular velocity for slow twitch muscle from Ranatunga
- 0.151 :RA tv at half max. angular velocity for slow twitch muscle from Ranatunga
- Any passive curve that is not accompanied by a curve from the literature (see the plots for details) is an educated guess.
Hip/Knee/Ankle: from Jackson and Anderson et al.
Lumbar Extension/Flexion: from Dolan et al.,Raschke et al., and Beimborn et al.
Shoulder 3 DoF torques: from Jackson and Kentel et al.
Elbow flexion/extension: from Kentel et al.
Wrist 3 DoF torques: from Jackson and Kentel et al.
Parameterized Curves used here vs. Literature
The curves used in this implementation are 2nd order 2-dimensional Bezier curves. The curves described in Anderson et al., Jackson, Kentel were not directly used because they are not continuous to the second derivative (a requirement for most gradient based optimization routines). There are some other detailed differences that might be of interest:
- Anderson et al.'s torque-velocity curve tends to large negative values for fast eccentric contractions. This is in contrast to the literature which says that at large eccentric contractions the torque-velocity curve (or the force-velocity-curve) tends to a value between 1.0 and 1.4.
- Anderson et al.'s torque-velcity curve for ankle extension did not cross the x-axis on the concentric side of the curve. This would endow the plantar flexors with super-human abilities. This error has been corrected by fitting a Bezier curve to a Hill-type curve that passes through the point where
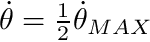
- Anderson et al.'s, Jackson, and Kentel et al. had discontinuities in the first derivative of the force velocity curve at
. While this follows Huxley's famous observations that the slope does discontinuously change at at
, this is not a phenomena that is not compatible with most optimal control formulations and thus this discontinuity is not present in the force velocity curves used in this model.
- Anderson et al. and Kentel et al.'s active-torque-angle curves can achieve negative values - this is obviously undesirable as it will allow a muscle to push.
Kentel et al.'s activation inhibition function does not always cross 1.0 for
, which means that
is not reached. This makes for a confusing model to use.
Coordinate Mapping
Every author chose a particular convention for measuring the angles of the hip, knee, ankle joint, shoulder, elbow, wrist and lumbar — see the figure for details. These conventions have all been mapped to the one used in the illustrations. You will need to use the figure, your model, and the constructors appropriately so that
- the joint angle of your model is correctly mapped to the fiber angle of the Millard2016TorqueMuscle;
- the sign of the muscle's output torque matches the sign associated with your model.
To map from your model's joint coordinates to the joint coordines used in this model (see the figure in the description) the followinq equation is used at the torque level

where fiberTorque is the torque produced by Anderson et al.'s curves, which is always positive. At the position level, the angles from your models joint angle to Anderson et al.'s joint angle (called fiberAngle) are mapped using

Internally the sign of the fiber velocity follows signOfJointTorque so that the signs of joint power and muscle power are consistent.
Strength Scaling: Anderson2007 Data Set
The leg strength (here we mean
) predicted by Anderson et al.'s curves should be taken as a good first approximation. While Anderson et al.'s data set is the most comprehensive in the literature, they only measured torques from active people: they did not include people at the extremes (both very weak, and very strong), nor did they include children. Finally, the torques produced by each subject were normalized by mSubjectMassInKg*subjectHeightInM*accelerationDueToGravity. Strength is a strange phenomena which is not nicely normalized by just these quantites, and so the strength predicted by Anderson et al.'s curves might not fit your subject even if they are represented in Anderson et al.'s data set.
Strength Scaling: Gymnast Data Set
The strength used in the Gymnast data set is fitted to an elite male gymnast. It goes without saying that an elite gymnast has strength proportions, and an absolute strength that are not typical. In the future it would be nice to have a function that could provide an educated guess about how to map Gymnast's strengths to that of another subject. For the moment I have no idea how to do this, nor am I aware of any works in the literature that can provide insight of how to do this. For now the whole-body Gymnast model should be viewed as being a representation of what is possible for a human, but not a typical human. At the present time the default strength settings of the Gymnast are not scaled by subject height, nor weight.
If you happen to know the maximum-isometric-active-torque (note this does not include the passive component) that your subject can produce, you can update the strength of the torque-muscle using the functions getMaximumActiveIsometricTorque(), and setMaximumActiveIsometricTorque().
Fitting to a Specific Subject
If you have recorded the motions and external forces acting on a subject during a movement of interest, you can make use of the TorqueMuscleFittingToolkit to adjust the MTG so that it is strong enough and flexible enough to allow a model of the subject to reproduce the experimental data. Please see the TorqueMuscleFittingToolkit class for details.
Limitations
This rigid-tendon torque muscle has some limitations that you should be aware of:
- There are no elastic tendons. That means that the mapping between the mechanical work done by this torque actuator will greatly differ from the positive mechanical work done by a torque actuator that includes an elastic tendon. This difference is greatest for those muscles with long tendons - namely the Achilles tendon in humans. If you are interested in fiber kinematics, fiber work, or metabolic energy consumption you cannot use this model especially for muscles that have long tendons.
- This model formulation predicts torque well, but does a poor job of predicting joint stiffness. In this model stiffness is given by the partial derivative of torque w.r.t. joint angle. Since the active-torque-angle curve fits a bell-shaped curve, it is possible to construct a torque muscle that has a region of physically impossible negative stiffness. Real muscle, in constrast, always has a positive stiffness even on the descending limb of the active-torque-angle curve (see Rassier et al. for details).
- Muscles that cross 2 joints (e.g. the hamstrings) produce coupled torques at both of those joints. In this model there is no coupling between joints. Furthermore, because of the lack of coupling the curves used here are only valid for the posture that Anderson et al., Jackson, and Kentel et al. used when they made their data collection. If you are interested in simulating postures that are very different from those described in by these authors then the results produced by this model should be treated as very rough.
- Because this is a joint-torque muscle, none of the joint contact forces predicted will come close to matching what is produced by line-type muscles. If you are interested in joint-contact forces you cannot use this model.
This simple model is a fast approximate means to constrain the joint torque developed in the body to something that is physiologically possible. That is it.
Units Although the figure in this description has angles in units of degrees, this is only to help intuitition: when using the model, use radians. This model uses MKS:
-Distance: m
-Angles: radians
-Angular velocity: radians/s
-Mass: kg
-Torque: Nm
-Time: second
-Power: Nm/second
Developer Notes
All of the numerical evaluations of this model take place in a few private stateless functions: -updateTorqueMuscleSummary -updTorqueMuscleSummaryCurveValues -updTorqueMuscleInfo -updInvertTorqueMuscleSummary If you want to change something fundamental about the mathematics of the model, then you have to update these functions.
References
- Anderson, D. E., Madigan, M. L., & Nussbaum, M. A. (2007). Maximum voluntary joint torque as a function of joint angle and angular velocity: model development and application to the lower limb. Journal of biomechanics, 40(14), 3105-3113.
- Beimborn, D. S., & Morrissey, M. C. (1988). A review of the literature related to trunk muscle performance. Spine, 13(6), 655-660.
- Christophy, M., Senan, N. A. F., Lotz, J. C., & O’Reilly, O. M. (2012). A musculoskeletal model for the lumbar spine. Biomechanics and Modeling in Mechanobiology, 11(1-2), 19-34.
- Dolan, P., A. F. Mannion, and M. A. Adams. Passive tissues help the back muscles to generate extensor moments during lifting. Journal of Biomechanics 27, no. 8 (1994): 1077-1085.
- Jackson, M.I. (2010). The mechanics of the Table Contact Phase of Gymnastics Vaulting. Doctoral Thesis, Loughborough University.
- Kentel, B.B., King, M.A., & Mitchell, S.R. (2011). Evaluation of a subject-specific torque-driven computer simulation model of one-handed tennis backhand ground strokes. Journal of Applied Biomechanics, 27(4),345-354.
- Millard, M., Uchida, T., Seth, A., & Delp, S. L. (2013). Flexing computational muscle: modeling and simulation of musculotendon dynamics. Journal of biomechanical engineering, 135(2), 021005.
- Németh, G., & Ohlsén, H. (1986). Moment arm lengths of trunk muscles to the lumbosacral joint obtained in vivo with computed tomography. Spine, 11(2), 158-160.
- Ranatunga, K. W. (1984). The force-velocity relation of rat fast-and slow-twitch muscles examined at different temperatures. The Journal of Physiology, 351, 517.
- Raschke, U., & Chaffin, D. B. (1996). Support for a linear length-tension relation of the torso extensor muscles: an investigation of the length and velocity EMG-force relationships. Journal of biomechanics, 29(12), 1597-1604.
- Rassier, D. E., Herzog, W., Wakeling, J., & Syme, D. A. (2003). Stretch-induced, steady-state force enhancement in single skeletal muscle fibers exceeds the isometric force at optimum fiber length. Journal of biomechanics, 36(9), 1309-1316.
Definition at line 922 of file Millard2016TorqueMuscle.h.